Whether you are a web developer, a development team leader or even a business owner - efficient development of applications is a clear benefit we all aim for. Choosing the right tools can make a significant difference in your productivity and code quality. While many IDEs are available, PHPStorm stands out as a powerful and feature-rich environment specifically designed for PHP developers.
But don’t get discouraged if you work on frontend - with a few extra plugins, you can add other languages, and most of the known Frameworks support you’re missing.
To help you get started with PHPStorm, we have gathered a list of tips and tricks, useful built-in features, favorite shortcuts, and useful plugins that will speed up your work. Even an experienced PHPStorm user might find new or long-forgotten tricks here, so take a few minutes and enjoy our PHPStorm Tips and tricks selection!
Built-in features
PHPStorm is at the very top among IDEs when it comes to out-of-the-box features. Here are the most important ones you get.
Code Completion and Auto-Completion
PHPStorm's Code Completion and Auto-Completion features are like having a coding copilot by your side, constantly suggesting the right code and helping you avoid common errors.
Here's how they work their magic:
- Time-Saving Typing: fill in long functions or variable names without risk of spelling errors
- Intelligent Suggestions: PHPStorm goes beyond simple suggestions. It analyzes your code context and provides intelligent recommendations based on your project's structure, language rules, and even your coding style.
- Error Prevention: Auto-Completion helps you avoid common errors by suggesting the correct syntax and parameters for functions and methods. It even highlights potential issues as you type, giving you immediate feedback and a chance to correct them before they become bigger problems.
With default PHPStormSetup you’ll start with Basic Auto-completion enabled. You can change that in Settings , Editor , General , Code Completion.
Basic completion (default)
So when you start typing a variable name, you’ll see suggestions that are available based on both caret position and your code.
Use the shortcut ^CTRL + SPACE or select Code , Code Completion , Basic from the main menu if you have disabled Auto-completion.
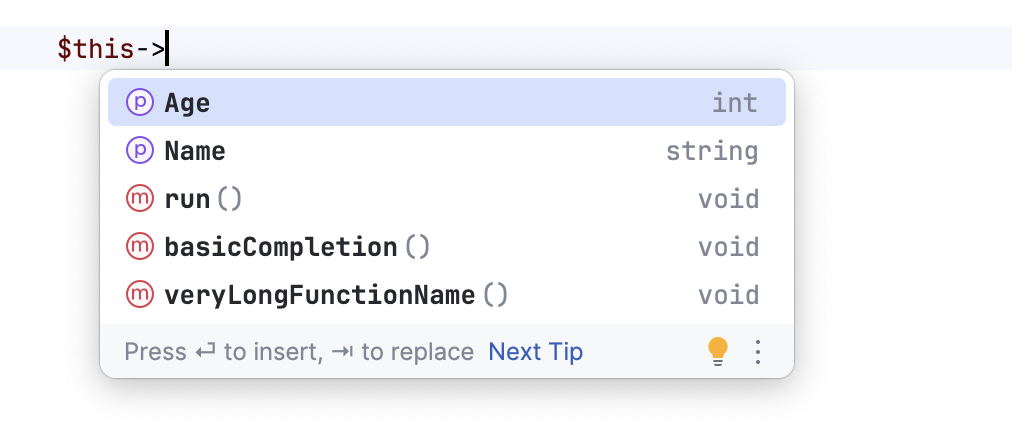
Type-matching completion
This option additionally checks for your types. It will limit the suggestions to ones matching your case. To use it you can press ^CTRL + OPT/ALT + SPACE or choose from the menu Code , Code Completion , Type-Matching.
What’s worth remembering is that you can switch between Basic and Types Completion at any time, even with an opened suggestions popup. Just use the proper shortcut to change the context of your suggestions.

Full completion features list
Basic and Type-matching completion are just the first, most commonly used features. In PHPStorm you’ll find much, much more, though:
- Statement completion
- Hippie completion
- Postfix completion
- Tags and attributes completion
- Machine-learning-assisted completion - thanks to which you can prioritize completion suggestions thanks to machine learning
- Full line code completion - which also uses machine learning
- Path completion (unavailable on macOS :( ) - which allows to fill paths in Browse windows
<div class="rtb-text-box is-blue-50"> Here is a full description of all code completion features</div>
Code and files navigation
PHPStorm’s deep understanding of your project allows it to provide one of the best code navigation systems we have out there.
Jumping between files
- Ctrl+Shift+N (Windows/Linux) or Cmd+Shift+O (macOS): This powerful shortcut brings up a search bar where you can type the name of any file you want to access.
- Recent Files: You can access recently opened files through the File > Recent Files menu or the Recent Files list in the Welcome Screen.
Jumping Between Classes:
- Ctrl+N (Windows/Linux) or Cmd+N (macOS): This shortcut opens a search bar for classes within your project. Start typing the class name, and PhpStorm will suggest matching options.
- Go to Declaration: Clicking on a class name in your code and pressing Ctrl+B (Windows/Linux) or Cmd+B (macOS) will take you directly to its declaration.
Jumping Between Methods and Functions
- Ctrl+Shift+Alt+N (Windows/Linux) or Cmd+Shift+Alt+N (macOS): This shortcut allows you to search for any symbol, including methods and functions.
- Go to Declaration: Similar to classes, you can click on a method or function name and press Ctrl+B (Windows/Linux) or Cmd+B (macOS) to jump to its declaration.
- Find Usages: To see where a method or function is used in your project, right-click on it and select Find Usages.
Navigation Commands and markers
- Go to Symbol: Use Ctrl+Alt+Shift+O (Windows/Linux) or Cmd+Alt+Shift+O (macOS) to navigate through the symbols in your project. This is useful for finding specific methods or variables.
- Change Markers: You can set markers in your code to quickly jump back to specific locations. To set a marker, click in the gutter next to the line number and select the desired marker type. You can then navigate between markers using Ctrl+Shift+F12 (Windows/Linux) or Cmd+Shift+F12 (macOS).
Other Tips:
- Structure View: The Structure View (View > Tool Windows > Structure or Alt+7) provides a hierarchical view of your code, allowing you to quickly navigate through classes, methods, and variables.
- File Structure Popup: Press Ctrl+F12 (Windows/Linux) or Cmd+F12 (macOS) to view a popup with the structure of the current file, making it easy to jump between different parts of the code.
- Search everywhere - Press SHIFT, SHIFT to open the search everywhere popup, it will look through classes, filenames, actions etc.
- Sticky lines pinned to the top in the editor: This feature allows you to keep important commands visible at the top of the terminal window, even as you scroll through the output.
Code folding - use CMD + SHIFT + - (CTRL SHIFT -) to fold all code in a file. Then use CMD + (CTRL +) or use arrows in the editor (next to line numbers) to expand folded code blocks one by one. You will get a nice, compact, and easy-to-read list of methods.

Refactoring
We all realize the importance of good-quality code. And when we talk about it first thing that comes to mind is refactoring.
PHPStorm also comes here with a set of great features to help you on your way:
- Use context (right click) refactoring popup or main Refactor menu to access it’s features
- Renaming - use Refactor , Rename option to change the name of the method, class, or variable. It will look for the variable occurrences based on your code structure and rename all relevant places. It can also look through comments and more.
- Extract Method - when you, e.g., have some assignment, simply refactor it with the Extract Method option. It will create the new method with your code and add proper. You’re done - two clicks and you have created the method out of the assignment.
ExtractVariable works similarly to the Extract Method.
First, mark an expression in your code.
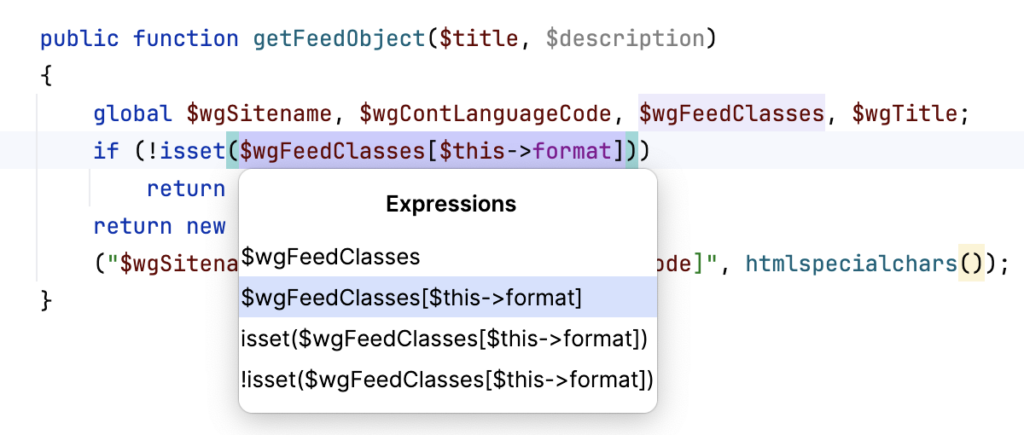
Debugging
- Then, use the Refactor , Extract variable option.
PHPStorm will create a new variable out of the expression and it will use it where it can.
If you have more occurrences, it will ask you to specify the scope of extraction so you can do it at the class level if necessary.
Debugging code is a very useful skill for developers. It allows them to examine the problem from a different perspective, freeze code execution, display the current state of the application, and more.
The most common Xdebug configuration requires three steps to get you started (or rather stopped ;) ):
- Install and configure Xdebug extension in PHP
- Add a debugging extension to your browser
- Configure PHPStorm to listen to incoming connections
Now open your PHPStorm editor and set a Breakpoint.

When you open your website, the code execution will stop.
Now you can use amazing debugging features like:
- Conditional breakpoints - add a condition for the breakpoint to work, e.g., in a loop to stop at certain values
- Variables inspections - display the state of variables in your code
- Step into, step over, and continue execution - follow the code as it executes step by step and find that place in the code you wanted
<div class="rtb-text-box is-blue-50"> For detailed configuration instructions, click here</div>
Version Control Integration
VCS is another out-of-the-box feature that is richly supported in the UI. It gives you a nice VCS window with branch graphs, integrated diffs, and other tools.
- Supports main version control shortcuts,
- Supports basic actions for all supported version control systems,
- Code review,
- Works with GitHub pull requests,
- Create, review, and comment on pull requests.
- Code editor VCS Annotations - in the left gutter by clicking VCS -> Git -> Annotate from the menu.
- Folders with VCS changes highlighting - enable File , Settings , Version Control , Confirmation , Highlight directories that contain modified files.
- Own Stash - it expends git’s stash functionality greatly and allows you to stash many commits for your branches
Local history
This one could save your life if you get in a mess.Just right click any file or folder in the project window and choose local history. PHPStorm tracks ALL local file changes. You can search through them, diff them and roll back to them (just like in git).

Project files management
Again there are lots and lots of features here. Try those tips:
- Scopes - Configurable via Settings , Appearance and Behavior , Scopes scopes allow you to better group your source files. Add Scopes for sources, external libraries, public directories. Later use the PHPStorm search features and narrow the results with those file scopes
- Exclude, set as Source or TestSource files / directories - use context menu on Directory or file to use scope features. A great tip is to Exclude your project’s external libraries to stop them from indexing by PHPStorm. This will speed up your environment.

Database tools
PHPStorm's integrated database tools offer a variety of features for working with almost all types of databases. The most important ones are as follows:
- Connect to any database, choose your schemas to work with,
- Query, create, and manage databases locally, on a server, or in the cloud
- View and modify data structures in the Database tool window or Query Consoles
- Full Webstorm and full Datagrip are already included within a single IDE.
- Import, export data
Terminal Integration
PHPStorm comes with an integrated terminal, a powerful tool that allows you to work with your command-line shell directly from within the IDE. Some of the more interesting features are:
- Full line code completion with local AI: This helps you write commands more quickly and accurately by suggesting completions as you type.
- Visual changes to each ran command being put in its own block: This feature makes it easier to see the output of each command and to navigate through the history of commands.
- Configure terminal keybindings - You can customize the shortcuts that are available in the Terminal tool window to suit your preferences.
<div class="rtb-text-box is-blue-50"> See the full terminal feature list here</div>
Testing
It’s unsurprising that PHPStorm also provides integration for PhpUnit and Behat, those famous testing tools.
For PHPUnit, IDE allows you to run tests directly from the interface, view test results, and debug tests. You can also use code completion and navigation features to write and maintain your tests more efficiently.
For Behat, we have support for running, viewing results, and debugging scenarios. You can also use code completion and navigation features to write and maintain your test.
<div class="rtb-text-box is-blue-50">You can find more detailed instructions here</div>
Essential plugins
If you still lack functionality, PHPStorm’s community provides many valuable plugins. We encourage you to find the ones best for your needs, but please take a look at those we’ve selected. You won’t regret it.
PHP Annotations
If you work in modern frameworks, you will probably be using the PHP annotation feature.
This uses commented DocBlocks to provide additional functionalities and attributes features.

Enable it if you work, for example, on Symfony projects (with described in this article Symfony plugin).
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
PHP Inspections (EA Extended)
If you work with code quality in mind, then install this Code Review and Static Code Analysis tool for PHP.
This plugin is designed to help you find defects, security issues, performance problems, and other potential issues in your PHP code.
Some of the features include:
- Code Inspections - static code analysis to identify potential issues in your code
- Code Completion - suggestions as you type, helping you write code more efficiently
- Code Navigation - navigate your codebase, quickly jump between files, classes, methods, and functions
- Code Refactoring
- Code Generation - creates for you getters and setters, constructors, and other common code patterns
- Code Documentation - great help in generating the docs
- Code Security - identify security vulnerabilities
- Code Performance - helps with identifying performance bottlenecks
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
PHP Toolbox
That’s another popular plugin you’ll like. It aims to improve the overall PhpStorm experience by providing a collection of tools and enhancements. Some of its most useful features include:
- Improved type hint variable completion,
- Better callable array completion and navigation
- Global string navigation for classes, methods, and functions
- Enhances core PhpStorm functionality like Find Duplicates or Generate Constructor IDE options (and more)
- Provides better auto-completion for libraries like PHPUnit, Behat, and Symfony.
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
Symfony Support
If you are working on a Symfony based project, you need this one!
The plugin enhances PHPStorm integration with Symfony, providing features like code completion, navigation, and debugging. The plugin also includes inspections, linemarkers, generators, and search everywhere features.
Remember to activate it per project!
Settings , Languages & Framework , PHP , Symfony > Enable Symfony support.
You can also use the nifty Autoconfigure button or tune settings further.
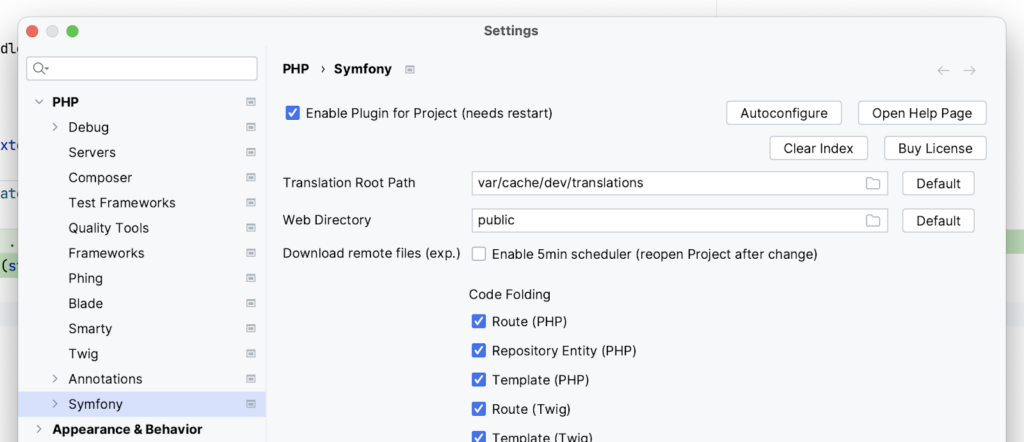
If you will be working with Symfony, we strongly recommend also next plugin, PHP Annotation.
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
GitToolBox
PHPStorm comes with a pretty great VCS integration. But if you work a lot with Git, and you probably do :), than consider extending the Git features with benefits like:
- Number of ahead, behind commits, and not committed changes in project view and status bar
- Commit message reference completion
- Commit message validation
- Branches cleanup - merged
- Integration with IDE Issue Navigation
- Automatic fetch at set intervals
- Push selected tags on current branch
- and more
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
PhpUnit Enhancement
Although PHPStorm comes with PhpUnit support when you write your tests you’ll be glad to use those extra features:
- Prophecy support.
- Mockery support:
- Referencing, autocompletion, refactoring
- Method highlighting
- Inspector to update legacy Mockery style
- Type providers for mock method functions
- Code navigation (go to declaration, find usages, etc.) and refactoring (rename methods);
- and more
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
String manipulation
This is a very versatile set of tools for operations on strings. Use it for:
- Case switching,
- Sorting,
- Filtering,
- Incrementing,
- Aligning to columns,
- Grepping,
- Escaping,
- Encoding
A nice trick with this plugin: Sort your translations yaml files with ease.
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
Key Promoter X
This plugin could greatly increase your IDE workflow by helping you learn and configure essential keyboard shortcuts for the actions you commonly use.
- Displays keyboard shortcut for your action every time you use mouse instead of keyboard
- Allows to add shortcuts to IDE windows for buttons that don’t have shortcuts and more efficiently manage frequently used popups
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >><div>
Tabnine
In the AI hype era we have to mention this plugin. Tabnine is an AI code assistant that helps you with real-time code completions, chat, and code generation in all the most popular coding languages and IDEs.
Just chat with it through PHPStorm about your code.
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
GitHub Copilot
I’m pretty sure you have heard about this one. This is another great AI based plugin written and trained on a huge library of GitHub’s code repositories.
<div class="rtb-text-box is-blue-100"> Learn more about the plugin >></div>
Rainbow Brackets and Indent Rainbow plugins
Whether you like those is a matter of preference, but those 2 plugins are worth at least trying out.
Rainbow Brackets is used to provide a nicer color syntax for your code. It colors variables, bracket pairs, xml tags and much more to increase the readability of your code.
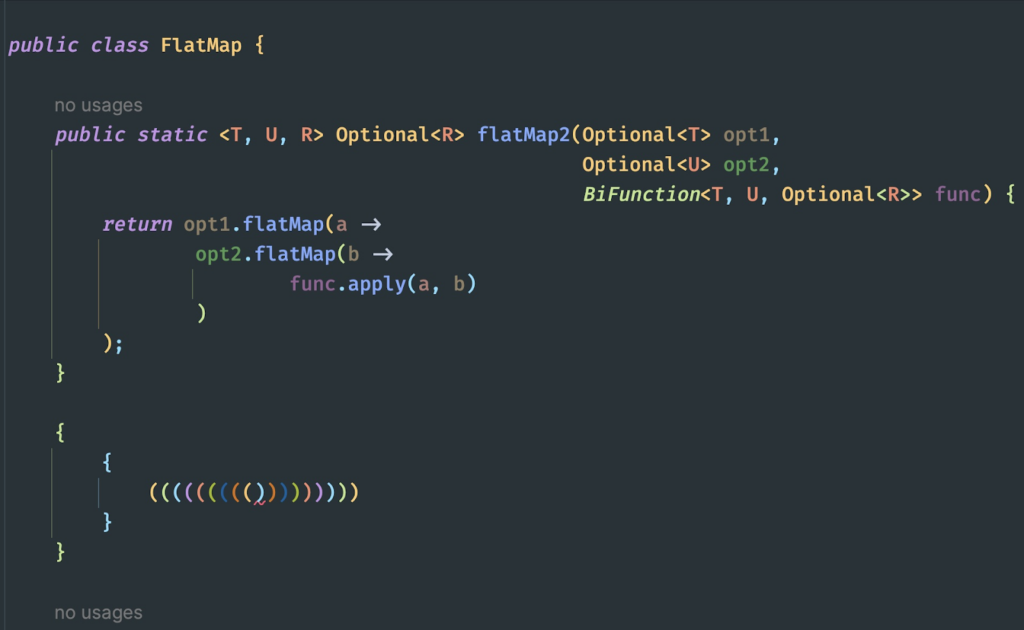

Indent Rainbow is a nice addition to this duet. It provides more readable indentation, colors your tabs and spaces, and makes reading code easier.
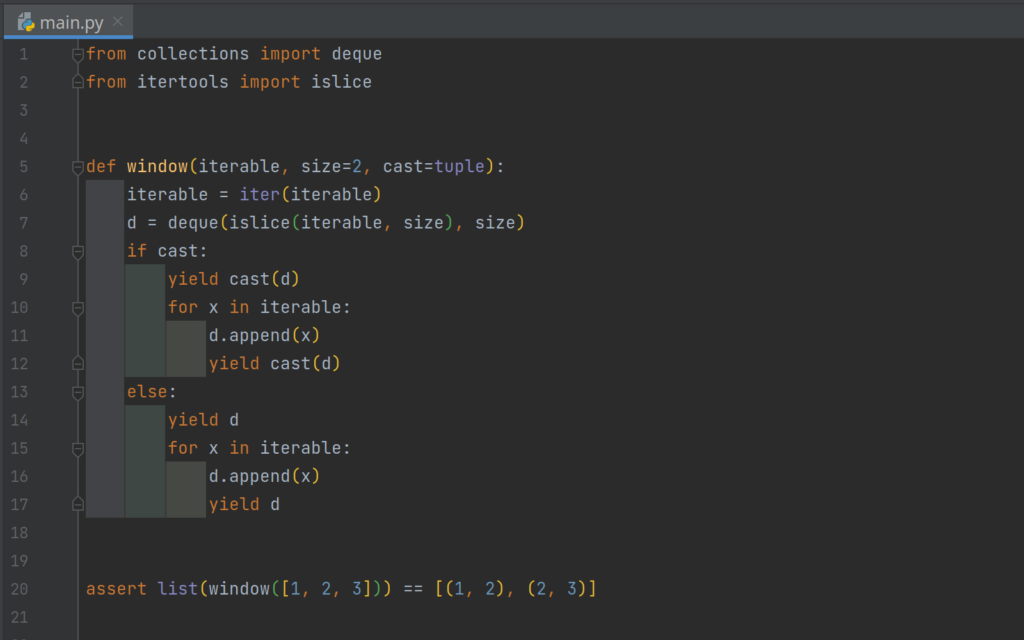
The keyboard shortcuts
A great starting point would be to have a PHPStorm Cheat Sheet printed out and laying on the table, next to your laptop. It’s a nice starting selection of PHPStorm shortcuts chosen by experienced users.
Here’s one you can grab right away, both for MacOS and Windows/Linux.
And if you are even more into Shortcuts, install the KeyPromoterX plugin described above - it helps in remembering shortcuts by displaying them every time you use the menu and mouse to do something.
Also, here’s an interesting and useful brief shortcuts list we’ve selected for you:
General shortcuts
- CMD + SHIFT + O (macOs) - Open file popup
- CMD + SHIFT + A (macOs) - Actions popup
- CMD + O (macOs) - go to Class
- SHIFT, SHIFT (macOs) - search everywhere popup
Code Editor Shortcuts
- SHIFT + F6 (macOs) - rename variable / method / class
- CTRL + V (macOs) / Ctrl+Alt+V - Introduce Variable
- OPT + ENTER - context actions
- CTRL + ENTER (macOs) / Alt + Insert - generate code menu
- CTRL + ALT +I - extract interface from class
- CTRL + OPT + O (macOs) / Ctrl+Alt+O - optimize imports (sort and remove unused imports)
- CTRL + T (macOs) / Ctrl+ Alt+Shift+T - Refactor this
- CMD + OPT + Z (macOs) / Ctrl+Alt+Z - rollback
VCS Shortcuts
- CTRL + V (macOs) / Alt+ ` - Version Control Operations popup
- CMD + K (macOs) - Commit
- CMD + SHIFT + K (macOs) - Push commit
- CMD + OPT + Z (macOs) / Ctrl+Alt+Z - rollback
Other Tools
We have already gone through the most important options for PHPStorm. But if you are hungry for more, you might also be interested in even more features you can find about more by yourself:
- Live Templates - use one of the predefined or create your own - interactive blocks of code ready to insert and fill with data
- Multi-line / multi-caret editing - place up to 500 carets and edit many lines at the same time
- Distraction-free view - hide most of the windows to focus on the code you work on
- Presentation mode - great for presentations, changes font sizes and UI elements for better readability on TVs and other devices
Conclusion
The feature list we’ve prepared is big. But it’s still nothing compared to what PHPStorm is capable of. After all, there’s a reason why it’s one of most valued IDEs on the market!
Remember about the features we’ve highlighted. Even get back to our post to refresh those tricks in a while, it won’t be a wasted time.